# canvas
# 1、简介
h5新增的标签,它可以用于动画、游戏画面、数据可视化、图片编辑以及实时视频处理等方面。
- 用途:绘制图表(Echars)、游戏、活动页面,动画小特效
- 相关框架
- 数据可视化:D3、antV、Echars
- 游戏:Eva.js
# 2、坐标体系
自上而下,自左从右,原点(0,0)在左上角,正常坐标系的第四象限
# 3、相关API
<canvas id="canvas" width="500" height="500"></canvas>
<script>
var canvas = document.getElementById('canvas');
var context = canvas.getContext('2d');
</script>
2
3
4
5
# 1、矩形API
context.fillRect(xPot, yPot, width, height),实心矩形,默认黑色
context.strokeRect(xPot, yPot, width, height),空矩形,默认黑色
# 2、线条API
context.beginPath();
context.moveTo(xPot, xPot); //设置起点
context.lineTo(xPot, xPot); //设置经过点
context.closePath(); //自动闭合回到起点
context.stroke(); //输出轮廓
context.fill(); //填充内容
# 3、圆弧API
context.arc(x, y, radius, startAngle, endAngle, anti-clockwise)
- x:圆的中心的x坐标
- y:圆的中心的y坐标
- radius:圆的半径
- startAngle:圆弧的开始角度,使用弧度表示
- endAngle:圆弧的结束角度
- antiClockwise:可选,顺时针还是逆时针,默认false,顺时针
var degree = 1; // 表示 1°
var radians = degree * (Math.PI / 180); // 0.0175弧度
//画实心圆
context.beginPath();
context.arc(250, 250, 200, 0, 2*Math.PI, false)
context.fill();
2
3
4
5
6
# 4、文本API
context.fillText(text, x, y) //实心字体
context.strokeText(text, x, y) //空心字体
context.font = "italic 60px serif" //字体(font-style)、字体大小、font-family
context.fillStyle = color
context.strokeStyle = "red #ff000 rgb(255, 0, 0) rgba(255, 0, 0, 0)"
context.lineWidth = 5 //设置线的宽度
# 5、插除
context.clearRect(x, y, width, height)
# 6、加载图像
context.drawImage(image, x, y, width, height)
var image = new Image()
image.src = "http://xxxx.svg"
image.onload = function(){//保证图片加载完成
context.drawImage(image, 50, 50, 120, 20);
}
2
3
4
5
# 7、动画
循环播放下一针画面,60Hz,使用requestAnimFrame
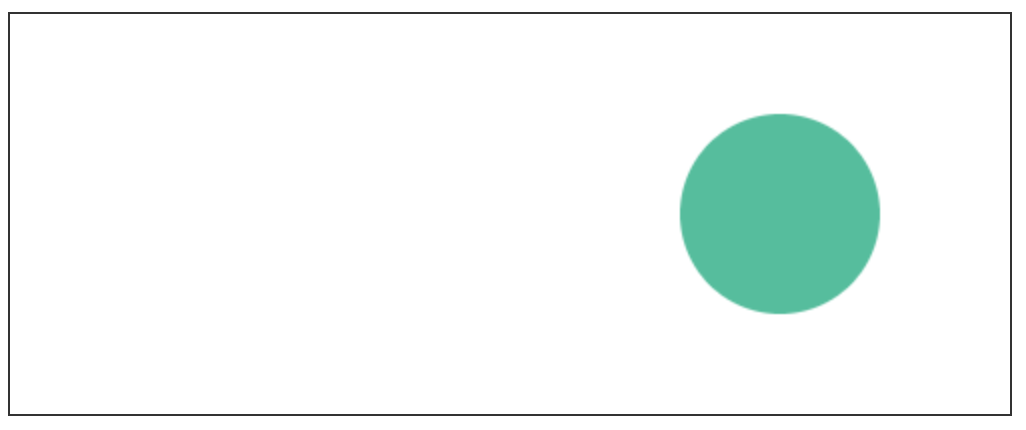
<html>
<head>
<title>canvas</title>
</head>
<body>
<canvas id="canvas" width="500" height="200" style="border: 1px solid #333333"></canvas>
<script>
var canvas = document.getElementById('canvas');
var context = canvas.getContext('2d');
//兼容定义requestAnimFrame
window.requestAnimFrame =
window.requestAnimationFrame ||
window.webkitRequestAnimationFrame ||
window.mozRequestAnimationFrame ||
window.oRequestAnimationFrame ||
window.msRequestAnimationFrame ||
function (callback) {
window.setTimeout(callback, 1000 / 30);
};
var circle = {
x: 500,
y: 100,
radius: 50,
direction: 'right',
move: function () {
if (this.direction === 'right') {
if (this.x <= 430) {
this.x += 5;
} else {
this.direction = 'left';
}
} else {
if (this.x >= 60) {
this.x -= 5;
} else {
this.direction = 'right';
}
}
},
draw: function () {
context.beginPath();
context.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
context.fillStyle = '#00c09b';
context.fill();
}
}
function animate() {
circle.move();
context.clearRect(0, 0, canvas.width, canvas.height);
circle.draw();
requestAnimationFrame(animate);
}
circle.draw();
animate();
</script>
</body>
</html>
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
# 8、键盘
监测键盘上下按键,移动。
碰撞检测:矩形检测左、右、顶、底侧,圆形检测距离小于半径和
if(!(rect2.x + rect2.width < rect1.x) &&
!(rect1.x + rect1.width < rect2.x) &&
!(rect2.y + rect2.height < rect1.y) &&
!(rect1.y + rect1.height < rect2.y)){
//矩形碰撞了
}
2
3
4
5
6